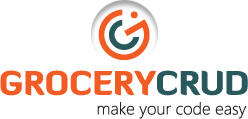
Do site do GroceryCRUD, um resumo do tutorial para iniciantes:
Executar a criação da tabela e preenchimento de registros de exemplo abaixo:
CREATE TABLE IF NOT EXISTS `employees` ( `employeeNumber` int(11) NOT NULL AUTO_INCREMENT, `lastName` varchar(50) NOT NULL, `firstName` varchar(50) NOT NULL, `extension` varchar(10) NOT NULL, `email` varchar(100) NOT NULL, `officeCode` varchar(10) NOT NULL, `file_url` varchar(250) CHARACTER SET utf8 NOT NULL, `jobTitle` varchar(50) NOT NULL, PRIMARY KEY (`employeeNumber`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=1703 ; INSERT INTO `employees` (`employeeNumber`, `lastName`, `firstName`, `extension`, `email`, `officeCode`, `file_url`, `jobTitle`) VALUES (1002, 'Murphy', 'Diane', 'x5800', 'dmurphy@classicmodelcars.com', '1', '', 'President'), (1056, 'Patterson', 'Mary', 'x4611', 'mpatterso@classicmodelcars.com', '1', '', 'VP Sales'), (1076, 'Firrelli', 'Jeff', 'x9273', 'jfirrelli@classicmodelcars.com', '1', '', 'VP Marketing'), (1088, 'Patterson', 'William', 'x4871', 'wpatterson@classicmodelcars.com', '6', '', 'Sales Manager (APAC)'), (1102, 'Bondur', 'Gerard', 'x5408', 'gbondur@classicmodelcars.com', '4', 'pdftest.pdf', 'Sale Manager (EMEA)'), (1143, 'Bow', 'Anthony', 'x5428', 'abow@classicmodelcars.com', '1', '', 'Sales Manager (NA)'), (1165, 'Jennings', 'Leslie', 'x3291', 'ljennings@classicmodelcars.com', '1', '', 'Sales Rep'), (1166, 'Thompson', 'Leslie', 'x4065', 'lthompson@classicmodelcars.com', '1', '', 'Sales Rep'), (1188, 'Firrelli', 'Julie', 'x2173', 'jfirrelli@classicmodelcars.com', '2', 'test-2.pdf', 'Sales Rep'), (1216, 'Patterson', 'Steve', 'x4334', 'spatterson@classicmodelcars.com', '2', '', 'Sales Rep'), (1286, 'Tseng', 'Foon Yue', 'x2248', 'ftseng@classicmodelcars.com', '3', '', 'Sales Rep'), (1323, 'Vanauf', 'George', 'x4102', 'gvanauf@classicmodelcars.com', '3', '', 'Sales Rep'), (1337, 'Bondur', 'Loui', 'x6493', 'lbondur@classicmodelcars.com', '4', '', 'Sales Rep'), (1370, 'Hernandez', 'Gerard', 'x2028', 'ghernande@classicmodelcars.com', '4', '', 'Sales Rep'), (1401, 'Castillo', 'Pamela', 'x2759', 'pcastillo@classicmodelcars.com', '4', '', 'Sales Rep'), (1501, 'Bott', 'Larry', 'x2311', 'lbott@classicmodelcars.com', '7', '', 'Sales Rep'), (1504, 'Jones', 'Barry', 'x102', 'bjones@classicmodelcars.com', '7', '', 'Sales Rep'), (1611, 'Fixter', 'Andy', 'x101', 'afixter@classicmodelcars.com', '6', '', 'Sales Rep'), (1612, 'Marsh', 'Peter', 'x102', 'pmarsh@classicmodelcars.com', '6', '', 'Sales Rep'), (1619, 'King', 'Tom', 'x103', 'tking@classicmodelcars.com', '6', '', 'Sales Rep'), (1621, 'Nishi', 'Mami', 'x101', 'mnishi@classicmodelcars.com', '5', '', 'Sales Rep'), (1625, 'Kato', 'Yoshimi', 'x102', 'ykato@classicmodelcars.com', '5', '', 'Sales Rep'), (1702, 'Gerard', 'Martin', 'x2312', 'mgerard@classicmodelcars.com', '4', '', 'Sales Rep');
Criar o arquivo Drive:\xampp\htdocs\codeigniter\application\views\our_template.php abaixo:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8" /> <?php foreach($css_files as $file): ?> <link type="text/css" rel="stylesheet" href="<?php echo $file; ?>" /> <?php endforeach; ?> <?php foreach($js_files as $file): ?> <script src="<?php echo $file; ?>"></script> <?php endforeach; ?> <style type='text/css'> body { font-family: Arial; font-size: 14px; } a { color: blue; text-decoration: none; font-size: 14px; } a:hover { text-decoration: underline; } </style> </head> <body> <!-- Beginning header --> <div> <a href='<?php echo site_url('examples/offices_management')?>'>Offices</a> | <a href='<?php echo site_url('examples/employees_management')?>'>Employees</a> | <a href='<?php echo site_url('examples/customers_management')?>'>Customers</a> | <a href='<?php echo site_url('examples/orders_management')?>'>Orders</a> | <a href='<?php echo site_url('examples/products_management')?>'>Products</a> | <a href='<?php echo site_url('examples/film_management')?>'>Films</a> </div> <!-- End of header--> <div style='height:20px;'></div> <div> <?php echo $output; ?> </div> <!-- Beginning footer --> <div>Footer</div> <!-- End of Footer --> </body> </html>Criar o arquivo Drive:\xampp\htdocs\codeigniter\application\controllers\main.php abaixo:<?php if ( ! defined('BASEPATH')) exit('No direct script access allowed'); class Main extends CI_Controller { function __construct() { parent::__construct(); /* Standard Libraries of codeigniter are required */ $this->load->database(); $this->load->helper('url'); /* ------------------ */ $this->load->library('grocery_CRUD'); } public function index() { echo "<h1>Welcome to the world of Codeigniter</h1>";//Just an example to ensure that we get into the function die(); } public function employees() { $this->grocery_crud->set_table('employees'); $output = $this->grocery_crud->render(); $this->_example_output($output); } function _example_output($output = null) { $this->load->view('our_template.php',$output); } } /* End of file main.php */ /* Location: ./application/controllers/main.php */Chamar a página http://localhost/codeigniter/index.php/main/employees, e, estando os serviços Apache e Mysql funcionando e o restante da configuração ok, teremos:
Nenhum comentário:
Postar um comentário